LV. Medium 🧐
https://leetcode.com/problems/gas-station/description/
Gas Station - LeetCode
Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview.
leetcode.com
문제
There are n gas stations along a circular route, where the amount of gas at the ith station is gas[i].
You have a car with an unlimited gas tank and it costs cost[i] of gas to travel from the ith station to its next (i + 1)th station. You begin the journey with an empty tank at one of the gas stations.
Given two integer arrays gas and cost, return the starting gas station's index if you can travel around the circuit once in the clockwise direction, otherwise return -1. If there exists a solution, it is guaranteed to be unique
Example 1:
Input: gas = [1,2,3,4,5], cost = [3,4,5,1,2]
Output: 3
Explanation:
Start at station 3 (index 3) and fill up with 4 unit of gas. Your tank = 0 + 4 = 4
Travel to station 4. Your tank = 4 - 1 + 5 = 8
Travel to station 0. Your tank = 8 - 2 + 1 = 7
Travel to station 1. Your tank = 7 - 3 + 2 = 6
Travel to station 2. Your tank = 6 - 4 + 3 = 5
Travel to station 3. The cost is 5. Your gas is just enough to travel back to station 3.
Therefore, return 3 as the starting index.
Example 2:
Input: gas = [2,3,4], cost = [3,4,3]
Output: -1
Explanation:
You can't start at station 0 or 1, as there is not enough gas to travel to the next station.
Let's start at station 2 and fill up with 4 unit of gas. Your tank = 0 + 4 = 4
Travel to station 0. Your tank = 4 - 3 + 2 = 3
Travel to station 1. Your tank = 3 - 3 + 3 = 3
You cannot travel back to station 2, as it requires 4 unit of gas but you only have 3.
Therefore, you can't travel around the circuit once no matter where you start.
Constraints:
- gas.length == n
- cost.length == n
- 1 <= n <= 105
- 0 <= gas[i], cost[i] <= 10^4
문제 해결법
각 station마다 gas - cost를 구해줬다.
그러면서 0번째 인덱스부터 gas - cost의 total 값을 구해줬다.
total값이 마이너스가 되면 시작할 수 없는 인덱스이기 때문에, 새로운 gas - cost > 0인 인덱스가 나오면 total를 0으로 리셋시켜줬다.
그리고 index를 저장해줬다.
gas - cost를 마지막까지 돌렸는데 total이 마이너스면 시작할 수 있는 인덱스가 없다는 뜻이기에 리턴 -1을 해줬다.
마지막에 나온 total를 가지고 다시 처음부터 index전까지 돌려 total이 중간에 마이너스로 가지 않고 for문을 다 돌면 index 리턴!
해결 코드
class Solution {
public:
int canCompleteCircuit(vector<int>& gas, vector<int>& cost) {
int size = gas.size();
int index = 0;
int total = 0;
for (int i = 0; i < size; ++i) {
gas[i] = gas[i] - cost[i];
if (total < 0 && gas[i] > 0) {
total = gas[i];
index = i;
}
else
total += gas[i];
}
if (total < 0)
return -1;
for (int i = 0; i < index; ++i) {
total += gas[i];
if (total < 0)
return -1;
}
return index;
}
};
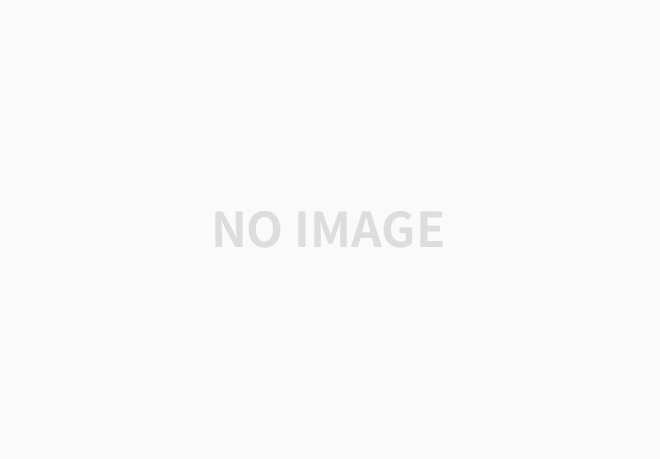
'Computer Science > 알고리즘' 카테고리의 다른 글
leetcode 1291) Sequential Digits (0) | 2022.01.25 |
---|---|
leetcode 520) Detect Capital (0) | 2022.01.25 |
leetcode 875) Koko Eating Bananas (0) | 2022.01.21 |
leetcode 849) Maximize Distance to Closest Person (0) | 2022.01.19 |
leetcode 142) Linked List Cycle II (0) | 2022.01.19 |
댓글