LV. Easy 😎
https://leetcode.com/problems/number-complement/
Number Complement - LeetCode
Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview.
leetcode.com
문제
The complement of an integer is the integer you get when you flip all the 0's to 1's and all the 1's to 0's in its binary representation.
- For example, The integer 5 is "101" in binary and its complement is "010" which is the integer 2.
Given an integer num, return its complement.
Example 1:
Input: num = 5
Output: 2
Explanation: The binary representation of 5 is 101 (no leading zero bits), and its complement is 010. So you need to output 2.
Example 2:
Input: num = 1
Output: 0
Explanation: The binary representation of 1 is 1 (no leading zero bits), and its complement is 0. So you need to output 0.
Constraints:
- 1 <= num < 2^31
문제 해결법
num를 2로 나누면서 나머지가 1이면 패스, 0이면 2^이진수의 자릿수를 곱해 num의 complement를 구한다
10진수를 2진수로 변환하는 법이 궁금하다면 아래 링크 클릭!
부동 소수점과 고정 소수점
이진 기수법 10진수에서는 10^n에 해당하는 수가 될 때마다 자릿수가 올라감 2진수에서는 2^n에 해당하는 수가 될 때마다 자릿수가 올라감 10진수 2진수 2 10 4 100 8 1000 9 1001 10진수를 2진수로 바꾸는
developer-eun-diary.tistory.com
해결 코드
#include <iostream>
#include <cmath>
class Solution {
public:
int findComplement(int num) {
int i = 0;
int complement = 0;
// get binary value and get complement value
while (num != 1) {
if (num % 2 == 0)
complement += pow(2, i);
num = num / 2;
i++;
}
return complement;
}
};
int main() {
Solution sol;
std::cout << sol.findComplement(1) << std::endl;
}
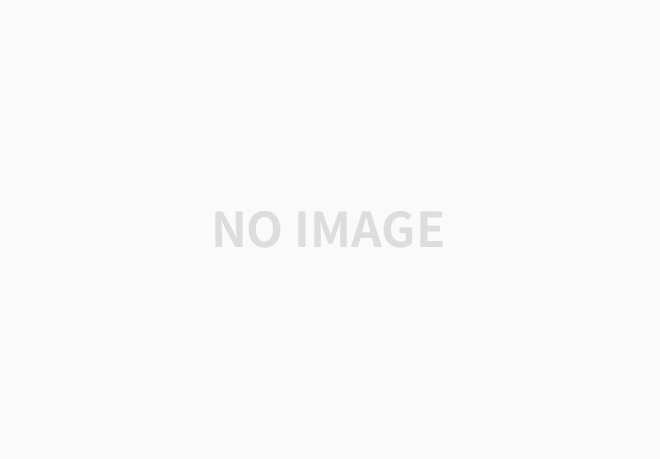
'Computer Science > 알고리즘' 카테고리의 다른 글
leetcode 416) Partition Equal Subset Sum (0) | 2022.01.04 |
---|---|
leetcode 221) Maximal Square (0) | 2022.01.04 |
leetcode 878) Nth Magical Number (0) | 2022.01.03 |
leetcode 790) Domino and Tromino Tiling (0) | 2022.01.02 |
leetcode 1217) Minimum Cost to Move Chips to The Same Position (0) | 2022.01.02 |
댓글