반응형
LV. Medium 🧐
https://leetcode.com/problems/swap-nodes-in-pairs/
Swap Nodes in Pairs - LeetCode
Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview.
leetcode.com
문제
Given a linked list, swap every two adjacent nodes and return its head. You must solve the problem without modifying the values in the list's nodes (i.e., only nodes themselves may be changed.)
Example 1:
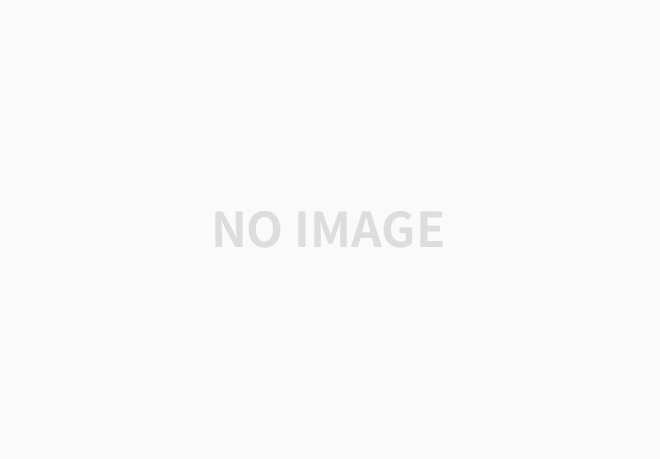
Input: head = [1,2,3,4]
Output: [2,1,4,3]
Example 2:
Input: head = []
Output: []
Example 3:
Input: head = [1]
Output: [1]
Constraints:
- The number of nodes in the list is in the range [0, 100].
- 0 <= Node.val <= 100
문제 해결법
head의 값을 처음부터 끝까지 한번 확인하면서 진행했다. O(1)
before의 값이 비워져 있으면 해당 노드를 before로 설정하고, before이 있으면 해당 노드와 before의 val값을 변경해주었다.
해결 코드
class Solution {
public:
ListNode* swapPairs(ListNode* head) {
ListNode *temp = head;
ListNode *before = 0;
while (temp) {
if (!before)
before = temp;
else {
int before_num = temp->val;
temp->val = before->val;
before->val = before_num;
before = 0;
}
temp = temp->next;
}
return head;
}
};
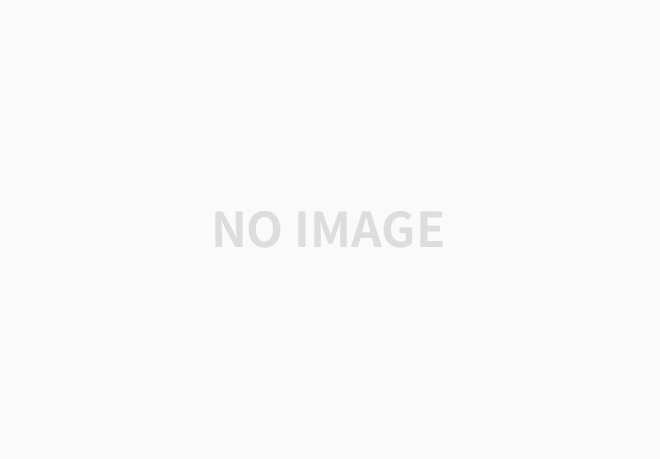
728x90
'Computer Science > 알고리즘' 카테고리의 다른 글
leetcode 402) Remove K Digits (0) | 2022.02.18 |
---|---|
leetcode 39) Combination Sum (0) | 2022.02.17 |
leetcode 136) Single Number (0) | 2022.02.15 |
leetcode 104) Maximum Depth of Binary Tree (0) | 2022.02.14 |
leetcode 78) Subsets (0) | 2022.02.13 |
댓글